Package opshin
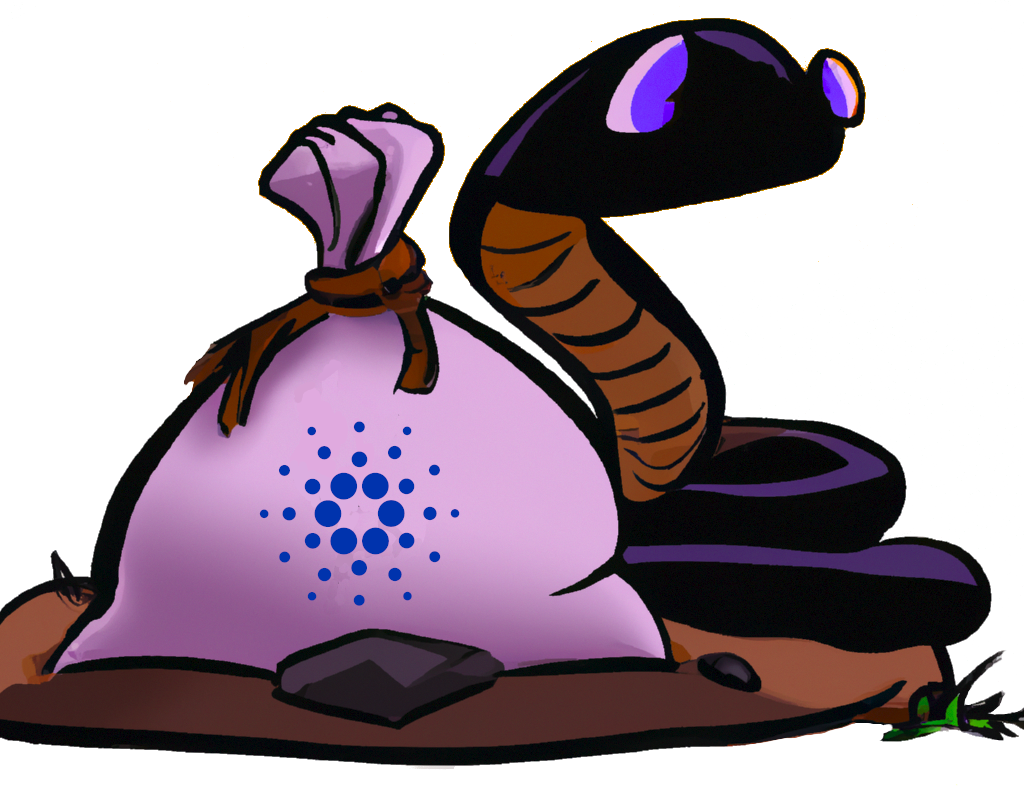
opshin
[](https://github.com/OpShin/opshin/actions/workflows/tests.yml)You are building what you want. Why not also build how you want?
This is an implementation of smart contracts for Cardano which are written in a strict subset of valid Python. The general philosophy of this project is to write a compiler that ensure the following:
If the program compiles then:
- it is a valid Python program
- the output running it with python is the same as running it on-chain.
Why opshin?
- 100% valid Python. Leverage the existing tool stack for Python, syntax highlighting, linting, debugging, unit-testing, property-based testing, verification
- Intuitive. Just like Python.
- Flexible. Imperative, functional, the way you want it.
- Efficient & Secure. Static type inference ensures strict typing and optimized code
Getting Started
Check out the OpShin Book for an introduction to this tool and details into writing smart contracts. This document will just outline the basic usage of the tool.
Installation
Install Python 3.8, 3.9, 3.10 or 3.11. Then run
python3 -m pip install opshin
Writing a Smart Contract
Check out the OpShin Book for an introduction to this tool and details into writing smart contracts.
Compiling
Write your program in python. You may start with the content of examples
.
Arguments to scripts are passed in as Plutus Data objects in JSON notation.
You can run any of the following commands
# Evaluate script in Python - this can be used to make sure there are no obvious errors
opshin eval spending examples/smart_contracts/assert_sum.py "{\"int\": 4}" "{\"int\": 38}" d8799fd8799f9fd8799fd8799fd8799f582055d353acacaab6460b37ed0f0e3a1a0aabf056df4a7fa1e265d21149ccacc527ff01ffd8799fd8799fd87a9f581cdbe769758f26efb21f008dc097bb194cffc622acc37fcefc5372eee3ffd87a80ffa140a1401a00989680d87a9f5820dfab81872ce2bbe6ee5af9bbfee4047f91c1f57db5e30da727d5fef1e7f02f4dffd87a80ffffff809fd8799fd8799fd8799f581cdc315c289fee4484eda07038393f21dc4e572aff292d7926018725c2ffd87a80ffa140a14000d87980d87a80ffffa140a14000a140a1400080a0d8799fd8799fd87980d87a80ffd8799fd87b80d87a80ffff80a1d87a9fd8799fd8799f582055d353acacaab6460b37ed0f0e3a1a0aabf056df4a7fa1e265d21149ccacc527ff01ffffd87980a15820dfab81872ce2bbe6ee5af9bbfee4047f91c1f57db5e30da727d5fef1e7f02f4dd8799f581cdc315c289fee4484eda07038393f21dc4e572aff292d7926018725c2ffd8799f5820746957f0eb57f2b11119684e611a98f373afea93473fefbb7632d579af2f6259ffffd87a9fd8799fd8799f582055d353acacaab6460b37ed0f0e3a1a0aabf056df4a7fa1e265d21149ccacc527ff01ffffff
# Compile script to 'uplc', the Cardano Smart Contract assembly
opshin compile spending examples/smart_contracts/assert_sum.py
Furthermore, you can add a shebang to the first line of the python file to indicate that it represents an opshin smart contract. You can choose from the following options:
- a general shebang:
#!opshin
, which representsopshin eval any
- or a more specific purpose:
#!/usr/bin/env -S opshin eval minting
By doing so, you can transform your python file to an executable: chmod +x your_file.py
and execute it with ./your_file.py
, which will run opshin eval any ./your_file.py
under the hood.
Deploying
The deploy process generates all artifacts required for usage with common libraries like pycardano, lucid and the cardano-cli.
# Automatically generate all artifacts needed for using this contract
opshin build spending examples/smart_contracts/assert_sum.py
See the tutorial by pycardano
for explanations how to build transactions with opshin
contracts.
API for Smart Contracts
The python interface offers a simple API to compile, load, apply parameters and evaluate smart contracts.
from opshin.builder import *
# Build a validator script from a python file that contains a validator function
contract = build("path/to/contract.py")
# You can apply parameters to the contract during compilation
contract = build("path/to/contract.py", arg1, arg2, arg3)
# Store the compilation artifacts in a folder
contract.dump("path/to/store")
# You can also load a compiled contract from a path
contract = load("path/to/store")
# And apply parameters after loading a contract
contract = contract.apply_parameters(arg1, arg2, arg3)
# The artifacts contain the compiled script, the policy ID and the addresses and blueprint
contract_addr = contract.mainnet_addr
contract_blueprint = contract.blueprint
Debugging artefacts
For debugging purposes, you can also run
# Compile script to 'uplc', and evaluate the script in UPLC (for debugging purposes)
opshin eval_uplc spending examples/smart_contracts/assert_sum.py "{\"int\": 4}" "{\"int\": 38}" d8799fd8799f9fd8799fd8799fd8799f582055d353acacaab6460b37ed0f0e3a1a0aabf056df4a7fa1e265d21149ccacc527ff01ffd8799fd8799fd87a9f581cdbe769758f26efb21f008dc097bb194cffc622acc37fcefc5372eee3ffd87a80ffa140a1401a00989680d87a9f5820dfab81872ce2bbe6ee5af9bbfee4047f91c1f57db5e30da727d5fef1e7f02f4dffd87a80ffffff809fd8799fd8799fd8799f581cdc315c289fee4484eda07038393f21dc4e572aff292d7926018725c2ffd87a80ffa140a14000d87980d87a80ffffa140a14000a140a1400080a0d8799fd8799fd87980d87a80ffd8799fd87b80d87a80ffff80a1d87a9fd8799fd8799f582055d353acacaab6460b37ed0f0e3a1a0aabf056df4a7fa1e265d21149ccacc527ff01ffffd87980a15820dfab81872ce2bbe6ee5af9bbfee4047f91c1f57db5e30da727d5fef1e7f02f4dd8799f581cdc315c289fee4484eda07038393f21dc4e572aff292d7926018725c2ffd8799f5820746957f0eb57f2b11119684e611a98f373afea93473fefbb7632d579af2f6259ffffd87a9fd8799fd8799f582055d353acacaab6460b37ed0f0e3a1a0aabf056df4a7fa1e265d21149ccacc527ff01ffffff
# Compile script to 'pluto', an intermediate language (for debugging purposes)
opshin compile_pluto spending examples/smart_contracts/assert_sum.py
Contributing
Developing and Technical Documentation
Generally, all contributions on the code side are very welcome. To get an overview over the architecture and idea behind OpShin, check out the Technical Documentation. A bug bounty has been set up and funded by Project Catalyst, which awards Github issue resolution wiht ADA rewards. This is a great opportunity to get involved and earn some ADA. Check out the detailed introduction to the bounty program for more information.
Sponsoring
You can sponsor the development of opshin through GitHub or Patreon or just by sending ADA. Drop me a message on social media and let me know what it is for.
- Patreon Support OpShin at Patreon to enjoy member benefits!
- GitHub Sponsor the developers of this project through the button "Sponsor" next to them
- ADA Donation in ADA can be submitted to
$opshin
oraddr1qyz3vgd5xxevjy2rvqevz9n7n7dney8n6hqggp23479fm6vwpj9clsvsf85cd4xc59zjztr5zwpummwckmzr2myjwjns74lhmr
.
Supporters
The main sponsor of this project is Inversion. Here is a word from them!
At Inversion, we pride ourselves on our passion for life and our ability to create exceptional software solutions for our clients. Our team of experts, with over a century of cumulative experience, is dedicated to harnessing the power of the Cardano blockchain to bring innovative and scalable decentralized applications to life. We've successfully built applications for NFT management, staking and delegation, chain data monitoring, analytics, and web3 integrations, as well as countless non-blockchain systems. With a focus on security, transparency, and sustainability, our team is excited to contribute to the Cardano ecosystem, pushing the boundaries of decentralized technologies to improve lives worldwide. Trust Inversion to be your go-to partner for robust, effective, and forward-thinking solutions, whether blockchain based, traditional systems, or a mix of the two.
They have recently started a podcast, called "Africa On Chain", which you can check out here: https://www.youtube.com/@africaonchain
Expand source code
#!/usr/bin/env python
# -*- coding: utf-8 -*-
"""
.. include:: ../README.md
"""
import warnings
__version__ = "0.24.2"
__author__ = "nielstron"
__author_email__ = "n.muendler@web.de"
__copyright__ = "Copyright (C) 2023 nielstron"
__license__ = "MIT"
__url__ = "https://github.com/OpShin/opshin"
try:
from .compiler import *
from .builder import *
except ImportError as e:
warnings.warn(ImportWarning(e))
Sub-modules
opshin.bridge
-
Bridging tools between uplc and opshin
opshin.builder
opshin.compiler
opshin.compiler_config
opshin.fun_impls
opshin.ledger
-
OpShin provides some helper classes that define concepts introduced in PlutusTx and used by the cardano node to encode data. In particular you find …
opshin.optimize
opshin.prelude
opshin.prelude_v3
opshin.rewrite
opshin.std
-
OpShin provides a few features in its standard libary. You can import modules from there (i.e. the
fractions
module) with … opshin.type_impls
opshin.type_inference
-
An aggressive type inference based on the work of Aycock [1]. It only allows a subset of legal python operations which allow us to infer the type of …
opshin.typed_ast
opshin.util